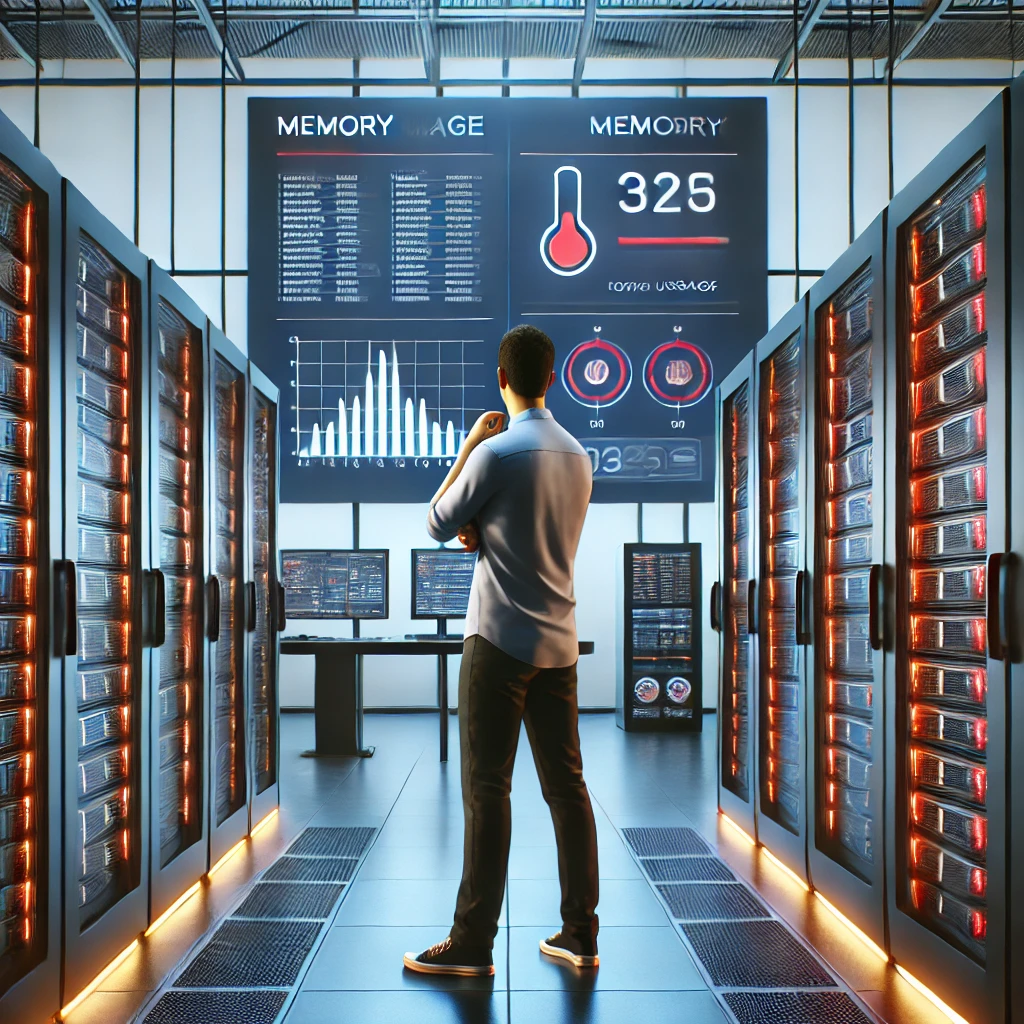
Node.js Memory Leaks: Detection and Resolution Guide (2025)
π§ Subscribe to JavaScript Insights
Get the latest JavaScript tutorials, career tips, and industry insights delivered to your inbox weekly.
Why Memory Leaks Matter in Node.js Applications (2025)
Memory leaks in Node.js applications lead to high memory usage, degraded performance, and crashes. In large-scale production systems, especially those serving thousands of concurrent requests, memory leaks can cause outages and downtime, impacting user experience and increasing infrastructure costs.
In 2025, Node.js v20.5.1 remains one of the most popular server-side runtimes. According to the Node.js User Survey 2025, over 42% of developers reported encountering memory issues at some point in production.
Memory leaks are difficult to detect because Node.js uses a garbage-collected language (JavaScript), which hides many low-level details. However, poor code practices, mishandled event listeners, and incorrect caching mechanisms often lead to persistent memory growth.
Who This Guide Helps
- Mid-Level and Senior Node.js Backend Developers
- Full-Stack Engineers maintaining backend systems
- DevOps Engineers monitoring Node.js applications
- Technical Architects designing scalable APIs
- Freelancers working on complex Node.js projects
Technical Solution
Step 1: Understand How Node.js Manages Memory
Node.js memory is divided into two main areas:
- V8 heap (managed by the JavaScript engine)
- Native memory (used by Node.js itself and third-party addons)
V8 heap is further split into:
- New Space (short-lived objects)
- Old Space (long-lived objects)
Use this command to check the memory limits for V8 (default is 2GB for 64-bit systems in Node.js v20.5.1):
Increase memory limit if necessary:
Step 2: Detect Memory Leaks in Node.js Applications
1. Monitor Memory Usage in Real-Time
Use process.memoryUsage()
to view memory statistics:
Expected behavior: memory should fluctuate but return to normal after garbage collection.
Signs of a memory leak: memory usage consistently grows over time without decreasing.
2. Use Node.js Built-In Profiler
Start the application with the inspector and collect heap snapshots:
Open Chrome DevTools:chrome://inspect
Take heap snapshots and compare them over time.
3. Use Clinic.js (v12.0.0)
Install Clinic.js:
Profile memory leaks with Clinic.js:
Review the report in clinic-doctor.html
.
4. Use Heapdump (v0.3.15)
Install Heapdump:
Trigger a snapshot programmatically:
Analyze the snapshot in Chrome DevTools.
Step 3: Common Causes of Node.js Memory Leaks
1. Global Variables and Unintentional References
Fix: implement cache size limits (e.g., LRU cache).
2. EventEmitter Memory Leaks
Too many event listeners trigger a warning and lead to leaks.
Fix: remove listeners or use once()
.
Or increase the listener limit (if needed):
3. Closures Retaining References
Fix: avoid unnecessary closures holding large objects.
Practical Implementation
Real-World Scenario: Memory Leak in an Express.js API
Problem
An Express.js (v5.0.0-beta.1) API showed steadily increasing heap memory in production.
Symptoms:
- Memory usage increased by ~50MB/hour
- Garbage collection did not reclaim memory
- Application restarted after hitting memory limits
Investigation
Added real-time memory monitoring:
Collected heap snapshots with Clinic.js and found unbounded growth in cached request objects.
Resolution
Replaced in-memory cache with an LRU cache implementation:
Result: heap usage stabilized, and no crashes occurred.
Testing & Validation
How to Verify the Fix Works
- Run automated load testing with Autocannon (v7.11.0):
Test the API endpoint:
- Monitor memory usage during the test
- Check for stabilized memory in the following metrics:
- Heap Used
- RSS (Resident Set Size)
- External Memory
- Validate no excessive garbage collections (GC logs):
Conclusion
Node.js memory leaks are tricky but manageable with the right tools and practices.
By following this guide, you can:
- Detect memory leaks early with real-time monitoring
- Profile and analyze leaks with heap snapshots
- Fix common causes like event listener leaks, unbounded caches, and closures
- Validate solutions through load testing and memory profiling tools
In 2025, scalable and reliable Node.js applications depend on proper memory management.
Take the time to profile, monitor, and refactor — your users (and servers) will thank you.